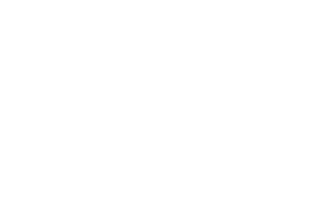
As you may know, whether it's not recommended or you should completely avoid it, having multiple elements with the same ID is wrong it's what we have class for :). Someone recently brought up the question on stackoverflow and it got me thinking, it's wrong yes but can it be done 3:D , the answer is...Yes. Don't get me wrong, it's terrible practice furthermore, less efficient than filtering by class as with this method you are filtering through every element in the DOM and checking against the ID attribute if it matches the user input but here is how you would achieve selecting multiple elements with the same ID. First, we create the method for document as below
document.getElementsById = function(ID){
}
now we have the new method getElementsByID, note this is different to getElementByID. Now we set up an array within the function, as after we loop through and push matching elements this will be returned.
document.getElementsById = function(ID){
var elements = [];
}
now we get onto looping through EVERY element :( , this is why we use class, and not ID.
document.getElementsById = function(ID){
var elements = [];
var allElements = document.getElementsByTagName("*");
for (var i = 0; i < allElements.length; ++i) {
}
}
to match ID we must first make a check to see if the element has the ID attribute set as below
document.getElementsById = function(ID){
var elements = [];
var allElements = document.getElementsByTagName("*");
for (var i = 0; i < allElements.length; ++i) {
if (typeof allElements[i].attributes.id !== "undefined") {
}
}
}
now that we have further narrowed the elements down to those with the attribute ID set we must check if the IDs match and if they do, push the element into the array elements,
document.getElementsById = function(ID){
var elements = [];
var allElements = document.getElementsByTagName("*");
for (var i = 0; i < allElements.length; ++i) {
if (typeof allElements[i].attributes.id !== "undefined") {
if (allElements[i].attributes.id.value == ID) {
elements.push(elem[i]);
}
}
}
}
now all we have to do is return the array
document.getElementsById = function(ID){
var elements = [];
var allElements = document.getElementsByTagName("*");
for (var i = 0; i < allElements.length; ++i) {
if (typeof allElements[i].attributes.id !== "undefined") {
if (allElements[i].attributes.id.value == ID) {
elements.push(elem[i]);
}
}
return elements;
}
No comments:
Post a Comment